★ Computers For Morons | Binary - Decimal ★
Heres your chance to share your own tutorials with the community. Just post them on here. If your lucky they may even be posted on the main site.
9 posts
Page 1 of 1
[center]The Basics of Binary and Decimal[/center]
Bytes represents a combination of 8 BITS, simplifying:
Now, moving to a more complex topic...
Before moving on to the conversion take a look to this:
Ok, enough with bullshit, its time to learn how you can convert those numbers to Binary:
Steps:
1) Effectuate successive divide operations by 2 until you get a 1.
2) Regroup the last quotient to all the rests of the division found by inversed order.
Example:
![Image]()
As you can see, the rests of that operations plus the last quotient combined shows you what's the Decimal "20" in Binary!
The way I got those rests was: for example, you can't divide 5 per 2, right? Then, subtract 1 to the 5 and store it, you'll get a 4, with the 4 you can now divide the 4 for 2, but always remember that rest. After completing the operation just add the "1" to the rests, and there you go!
In the operation above:
For example, we're trying to convert 1001 to Decimal...
For a better understanding:
Pick the digit number X from the combination and represent the potency of base 2 and index X-1
That isn't enough! To truly converting it to Decimal you'll have to add the partial multiplications done between the digit and the potency attributed to it...
We're trying to convert "10100" to Decimal:
10100(2) = 20(10)
1 x 24 + 0 x 23 + 1 x 22 + 0 + 0 x 20
After doing that operation we'll get the number "20"
It's a little hard to understand at the beginning, but after training some operations to convert binary to decimal you'll have mastered it.
This may help you in future applications you may want to do.
- What is a Binary that you mentioned in the title?
- What are the BITS and Bytes?
Bytes represents a combination of 8 BITS, simplifying:
Code: Select all
Bytes are also simplified with Kilobytes, Megabytes, Gigabytes and Terabytes.
1 Byte = 8 BITS
2 Bytes = 16 BITS
3 Bytes = 24 BITS
(...)
Code: Select all
1 Byte = 8 BITs ----> 256 Possible Combinations of 1 and 01 Kilobyte (KB) = 1024 Bytes
1 Megabyte (MB) = 1024 KBytes
1 Gigabyte (GB) = 1024 MByte
1 Terabyte (TB) = 1024 GByte
Now, moving to a more complex topic...
- Converting Decimal to Binary
Before moving on to the conversion take a look to this:
Code: Select all
Basically there are only 10 Decimal Digits existing in computer's language. 10, 11, 12, etc are combinations of the decimal digits, for example 11 is two ones (1) combined... In the table above you can see the representation of each one of the 10 decimal numbers in BITS.0 = 0000
1 = 0001
2 = 0010
3 = 0011
4 = 0100
5 = 0101
6 = 0110
7 = 0111
8 = 1000
9 = 1001
Ok, enough with bullshit, its time to learn how you can convert those numbers to Binary:
Steps:
1) Effectuate successive divide operations by 2 until you get a 1.
2) Regroup the last quotient to all the rests of the division found by inversed order.
Example:
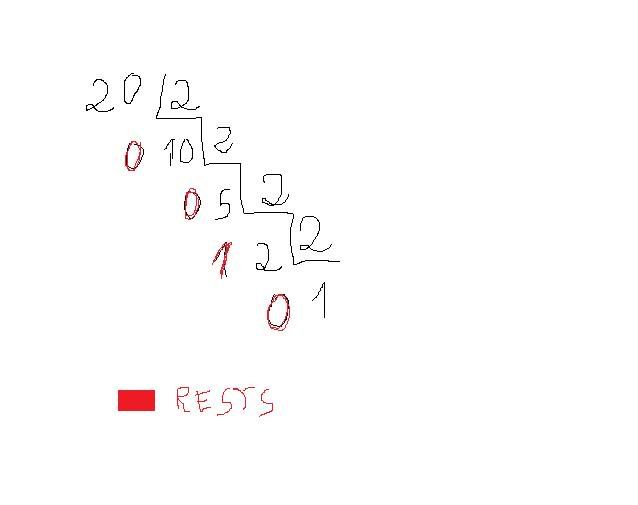
As you can see, the rests of that operations plus the last quotient combined shows you what's the Decimal "20" in Binary!
The way I got those rests was: for example, you can't divide 5 per 2, right? Then, subtract 1 to the 5 and store it, you'll get a 4, with the 4 you can now divide the 4 for 2, but always remember that rest. After completing the operation just add the "1" to the rests, and there you go!
In the operation above:
Code: Select all
I hope you understood!20(10) = 10100(2)
- Converting Binary to Decimal
For example, we're trying to convert 1001 to Decimal...
Code: Select all
That makes sense, doesn't it?- Pick the first Digit of that combination and represent it as a potency with base 2 and index 1
- Pick the second Digit of the combination and represent it as a potency with base 2 and index 0
- Pick the third Digit of the combination and represent it as a potency with base 2 and index 0
- Pick the fourth Digit of the combination and represent it as a potency with base 2 and index 1
For a better understanding:
Pick the digit number X from the combination and represent the potency of base 2 and index X-1
That isn't enough! To truly converting it to Decimal you'll have to add the partial multiplications done between the digit and the potency attributed to it...
We're trying to convert "10100" to Decimal:
10100(2) = 20(10)
1 x 24 + 0 x 23 + 1 x 22 + 0 + 0 x 20
After doing that operation we'll get the number "20"
It's a little hard to understand at the beginning, but after training some operations to convert binary to decimal you'll have mastered it.
This may help you in future applications you may want to do.
I might understand this when I get older, you lost me at the "Ok, enough with bullshit, its time to learn how you can convert those numbers to Binary:" and under :P
I like to make the computer work out the bits and bytes for me
Code: Select all
And this converts the binary string back to a number again:
Dim number As Int32 = 510 'example
Dim bytes As Byte() = BitConverter.GetBytes(number)
MsgBox("Your number takes up " & bytes.Length & " bytes")
Dim bits As BitArray = New BitArray(bytes)
MsgBox("That's " & bits.Length & " bits")
Dim readable As String = ""
Dim time As Integer = 0
For i As Integer = 0 To bits.Length - 1
time += 1
Dim temp As Integer = Convert.ToInt32(bits(i)) 'just used to store a 1 or 0
readable += temp.ToString()
If time = 8 Then
time = 0
readable += " " 'seperator per byte
End If
Next
MsgBox(readable)
Code: Select all
Dim readable As String = "01111111 10000000 00000000 00000000"
Dim strbytes As String() = readable.Split(" "c)
Dim bits As BitArray = New BitArray(strbytes.Length * 8)
Dim bitpos As Integer = 0
For i As Integer = 0 To strbytes.Length - 1
For i2 As Integer = 0 To 7
bits(bitpos) = strbytes(i)(i2).ToString()
bitpos += 1
Next
Next
Dim bytes(strbytes.Length - 1) As Byte
bits.CopyTo(bytes, 0)
Dim number As Int32 = BitConverter.ToInt32(bytes, 0)
MsgBox(number)
how would i convert from binary to decimal? (in vb)
You can use the above code but just change Int32 to Single.
Nery wrote: Ok, enough with bullshit.ENOUGH WITH THIS WHAT?!
9 posts
Page 1 of 1
Copyright Information
Copyright © Codenstuff.com 2020 - 2023